官方文档:https://cloud.tencent.com/document/product/436/10199
首先导入依赖
1 2 3 4 5 6 7 8 9 10 11 12
| <dependency> <groupId>com.qcloud</groupId> <artifactId>cos_api</artifactId> <version>5.6.89</version> </dependency>
<dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.1</version> </dependency>
|
创建一个 FileServer 类,处理上传逻辑
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| package com.szx.boot03tengxunyun.server;
import com.qcloud.cos.COSClient; import com.qcloud.cos.ClientConfig; import com.qcloud.cos.auth.BasicCOSCredentials; import com.qcloud.cos.auth.COSCredentials; import com.qcloud.cos.exception.CosClientException; import com.qcloud.cos.exception.CosServiceException; import com.qcloud.cos.http.HttpProtocol; import com.qcloud.cos.model.*; import com.qcloud.cos.region.Region; import com.szx.boot03tengxunyun.bean.CosBean; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component; import org.springframework.stereotype.Service; import org.springframework.web.multipart.MultipartFile;
import java.io.File; import java.io.IOException; import java.text.SimpleDateFormat; import java.util.Date; import java.util.List;
@Service public class FileServer { String secretId = ""; String secretKey = ""; String filePath = "https://szx-bucket1.oss-cn-hangzhou.aliyuncs.com/"; String bucketName = "blogimages-1257342648";
COSCredentials cred = new BasicCOSCredentials(secretId, secretKey); Region region = new Region("ap-shanghai"); ClientConfig clientConfig = new ClientConfig(region);
public String upload(MultipartFile multipartFile) throws IOException { String filename = multipartFile.getOriginalFilename(); clientConfig.setHttpProtocol(HttpProtocol.https); COSClient cosClient = new COSClient(cred, clientConfig);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); String[] fileNames= filename.split("\\."); String name = fileNames[0]+ sdf.format(new Date())+ filename.substring(filename.lastIndexOf("."), filename.length()); String key = "javaUpload/" + name; PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, key, multipartFile.getInputStream(),null); cosClient.putObject(putObjectRequest); return filePath + key; } }
|
封装一个 msg 类,用来返回上传成功返回的数据信息
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| package com.szx.boot03tengxunyun.bean;
import java.util.HashMap;
public class Msg { int code; String message; HashMap<String,Object> data = new HashMap<>();
public static Msg success(){ Msg msg = new Msg(); msg.setCode(200); msg.setMessage("成功"); return msg; }
public static Msg error(){ Msg msg = new Msg(); msg.setCode(500); msg.setMessage("失败"); return msg; }
public Msg add(String key,Object data){ this.getData().put(key,data); return this; }
public int getCode() { return code; }
public void setCode(int code) { this.code = code; }
public String getMessage() { return message; }
public void setMessage(String message) { this.message = message; }
public HashMap<String, Object> getData() { return data; }
public void setData(HashMap<String, Object> data) { this.data = data; }
public Msg(int code, String message, HashMap<String, Object> data) { this.code = code; this.message = message; this.data = data; }
public Msg() { } }
|
编写一个 FileController,调用 FileServer 中的 upload 方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| @RestController public class FileController { @Autowired FileServer fileServer;
@RequestMapping(value = "/upload",method = RequestMethod.POST) public Msg uploadFile(@RequestParam("file") MultipartFile multipartFile){ try { String filePath = fileServer.upload(multipartFile); return Msg.success().add("fileUrl",filePath); } catch (IOException e) { e.printStackTrace(); } return Msg.error(); } }
|
然后前端通过 post 方式调用 /upload 接口,同时传递文件过来。前端代码如下,使用了 ElementUI 中的文件上传组件
1 2 3 4 5 6 7 8
| <template> <div> <el-upload class="upload-demo" drag action="/upload" multiple> <i class="el-icon-upload"></i> <div class="el-upload__text">将文件拖到此处,或<em>点击上传</em></div> </el-upload> </div> </template>
|
运行效果:
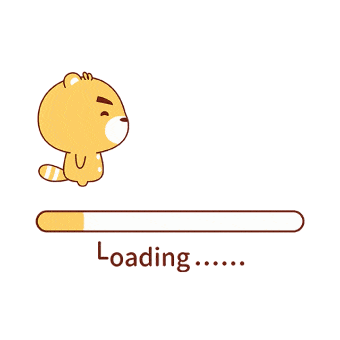
登录腾讯云控制台,访问对象存储模块,查看 javaUpload 文件夹下的内容
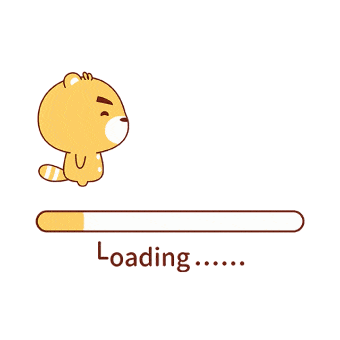
可以看到文件成功上传到云端